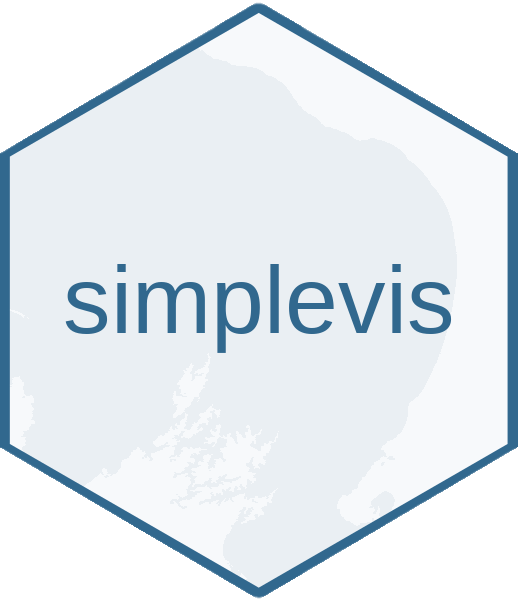
DEPREVATED. Violin ggplot that is coloured and facetted.
Source:R/gg_violin.R
gg_violin_col_facet.Rd
DEPREVATED. Violin ggplot that is coloured and facetted.
Usage
gg_violin_col_facet(
data,
x_var,
y_var = NULL,
col_var,
facet_var,
pal = NULL,
pal_na = "#7F7F7F",
pal_rev = FALSE,
alpha_fill = 1,
alpha_line = 1,
size_line = 0.5,
width = 0.75,
title = NULL,
title_wrap = 80,
subtitle = NULL,
subtitle_wrap = 80,
x_expand = ggplot2::waiver(),
x_labels = snakecase::to_sentence_case,
x_na_rm = FALSE,
x_rev = FALSE,
x_title = NULL,
x_title_wrap = 50,
y_zero_mid = FALSE,
y_breaks_n = 3,
y_expand = c(0, 0),
y_labels = scales::label_comma(),
y_title = NULL,
y_title_wrap = 50,
y_zero = FALSE,
y_zero_line = NULL,
col_labels = snakecase::to_sentence_case,
col_legend_none = FALSE,
col_na_rm = FALSE,
col_rev = FALSE,
col_title = NULL,
col_title_wrap = 25,
facet_labels = snakecase::to_sentence_case,
facet_na_rm = FALSE,
facet_ncol = NULL,
facet_nrow = NULL,
facet_rev = FALSE,
facet_scales = "fixed",
caption = NULL,
caption_wrap = 80,
theme = gg_theme(y_grid = TRUE),
model_scale = "area",
model_bw = "nrd0",
model_adjust = 1,
model_kernel = "gaussian",
model_trim = TRUE
)
Arguments
- data
A data frame in a structure to be transformed to density statistics. Required input.
- x_var
Unquoted categorical variable to be on the x scale (i.e. character, factor, logical). Required input.
- y_var
Generally an unquoted numeric variable to be on the y scale.
- col_var
Unquoted categorical variable to colour the fill of the boxes. Required input.
- facet_var
Unquoted categorical variable to facet the data by. Required input.
- pal
Character vector of hex codes.
- pal_na
The hex code or name of the NA colour to be used.
- pal_rev
Reverses the palette. Defaults to FALSE.
- alpha_fill
The opacity of the fill. Defaults to 1.
- alpha_line
The opacity of the outline. Defaults to 1.
- size_line
The size of the outlines of violins. Defaults to 0.5.
- width
Width of boxes. Defaults to 0.75.
- title
Title string.
- title_wrap
Number of characters to wrap the title to. Defaults to 75.
- subtitle
Subtitle string.
- subtitle_wrap
Number of characters to wrap the subtitle to. Defaults to 75.
- x_expand
A vector of range expansion constants used to add padding to the x scale, as per the ggplot2 expand argument in ggplot2 scales functions.
- x_labels
A function or named vector to modify x scale labels. If NULL, categorical variable labels are converted to sentence case. Use ggplot2::waiver() to keep x labels untransformed.
- x_na_rm
TRUE or FALSE of whether to include x_var NA values. Defaults to FALSE.
- x_rev
For a categorical x variable, TRUE or FALSE of whether the x variable variable is reversed. Defaults to FALSE.
- x_title
X scale title string. Defaults to NULL, which converts to sentence case with spaces. Use "" if you would like no title.
- x_title_wrap
Number of characters to wrap the x title to. Defaults to 50.
- y_zero_mid
For a numeric y variable, add balance to the y scale so that zero is in the centre of the y scale.
- y_breaks_n
For a numeric or date x variable, the desired number of intervals on the x scale, as calculated by the pretty algorithm. Defaults to 4.
- y_expand
A vector of range expansion constants used to add padding to the y scale, as per the ggplot2 expand argument in ggplot2 scales functions.
- y_labels
A function or named vector to modify y scale labels. Use ggplot2::waiver() to keep y labels untransformed.
- y_title
y scale title string. Defaults to NULL, which converts to sentence case with spaces. Use "" if you would like no title.
- y_title_wrap
Number of characters to wrap the y title to. Defaults to 50.
- y_zero
For a numeric y variable, TRUE or FALSE of whether the minimum of the y scale is zero. Defaults to TRUE.
- y_zero_line
For a numeric y variable, TRUE or FALSE whether to add a zero reference line to the y scale. Defaults to TRUE if there are positive and negative values in y_var. Otherwise defaults to FALSE.
- col_labels
A function or named vector to modify colour scale labels. Defaults to snakecase::to_sentence_case. Use ggplot2::waiver() to keep colour labels untransformed.
- col_legend_none
TRUE or FALSE of whether to remove the legend.
- col_na_rm
TRUE or FALSE of whether to include col_var NA values. Defaults to FALSE.
- col_rev
TRUE or FALSE of whether the colour scale is reversed. Defaults to FALSE.
- col_title
Colour title string for the legend. Defaults to NULL, which converts to sentence case with spaces. Use "" if you would like no title.
- col_title_wrap
Number of characters to wrap the colour title to. Defaults to 25. Not applicable where mobile equals TRUE.
- facet_labels
A function or named vector to modify facet scale labels. Defaults to converting labels to sentence case. Use ggplot2::waiver() to keep facet labels untransformed.
- facet_na_rm
TRUE or FALSE of whether to include facet_var NA values. Defaults to FALSE.
- facet_ncol
The number of columns of facetted plots.
- facet_nrow
The number of rows of facetted plots.
- facet_rev
TRUE or FALSE of whether the facet variable variable is reversed. Defaults to FALSE.
- facet_scales
Whether facet_scales should be "fixed" across facets, "free" in both directions, or free in just one direction (i.e. "free_x" or "free_y"). Defaults to "fixed".
- caption
Caption title string.
- caption_wrap
Number of characters to wrap the caption to. Defaults to 80.
- theme
A ggplot2 theme.
- model_scale
Per ggplot2::geom_violin, if "area" (default), all violins have the same area (before trimming the tails). If "count", areas are scaled proportionally to the number of observations. If "width", all violins have the same maximum width.
- model_bw
The bw argument of the stats::density function. Defaults to "nrd0".
- model_adjust
The adjust argument of the stats::density function. Defaults to 1.
- model_kernel
The kernel argument of the stats::density function. Defaults to "gaussian".
- model_trim
TRUE or FALSE of whether to trim the tails. Defaults to FALSE.
Examples
library(simplevis)
library(palmerpenguins)
penguins %>%
dplyr::mutate(year = as.character(year)) %>%
gg_violin_col_facet(x_var = year,
y_var = body_mass_g,
col_var = sex,
facet_var = species,
col_na_rm = TRUE,
x_labels = function(x) stringr::str_sub(x, 3, 4))